Appendices
Appendix A: DLLs used to support coupon projects
The DDLs used to support this functionality are listed below and should reside in the primary database and schema that RPI is configured to use. Using an Auxiliary database may also be an option, but that has not been validated or tested at this point.
Coupon pool
-- Coupon Pool Table Create Statement
CREATE TABLE [dbo].[RPI_COUPON_POOL](
[promotion_code] [varchar](100) NOT NULL,
[coupon_code] [varchar](100) NOT NULL,
[display_name] [varchar](100) NOT NULL,
[max_uses] [int],
[start_date] [datetime],
[expiration_date] [datetime],
[allocated] [int],
[allocation_ts] [datetime],
) ON [PRIMARY]
GO
Field reference
Field name | Description |
---|---|
| This is the name of the promotion that the codes are associated to. This will be used to define a view to support using as the source of the metadata values. |
| This is the coupon code itself and should be unique in the table. |
| This would be used if you had a name that you wanted to display along with the coupon itself. Some examples are: |
|
This field is not a part of the sample coupon allocation project, but could be implemented if that is a requirement. This is used to support multi-use codes and specifies what the limit of uses is. |
|
This field is not a part of the sample coupon allocation project, but could be implemented if that is a requirement. Start date can be used to define when a coupon is valid. |
|
This field is not a part of the sample coupon allocation project, but could be implemented if that is a requirement. Expiration Date can be used to define when a coupon is invalid. |
| This is field is set to |
| This is the timestamp of when the coupon was allocated. |
Coupon assignment
Keep in mind that this example table uses names like resolution_key_int01
to support any resolution keys that are integers and used as a resolution to which coupons are getting assigned. We have a similar set for strings (resolution_key_str01
). These generic names could be used or you could rename these columns to the specific names of the resolution keys, if that makes things easier to understand. You would just need to make sure that the RPDM project is updated to support the name changes.
Using multiple resolutions for assigning coupons is possible, but requires some additional configuration in RPI and RPDM.
CREATE TABLE [dbo].[RPI_COUPON_ASSIGNMENT](
[coupon_assignment_id] [int] IDENTITY(1,1) NOT NULL,
[promotion_code] [varchar](100) NOT NULL,
[coupon_code] [varchar](100) NOT NULL,
[coupon_type] [varchar](100) NOT NULL,
[RPIResolutionKey] [varchar](100),
[resolution_key_int01] [bigint],
[resolution_key_int02] [bigint],
[resolution_key_int03] [bigint],
[resolution_key_str01] [varchar](100),
[resolution_key_str02] [varchar](100),
[resolution_key_str03] [varchar](100),
[insert_ts] [varchar](100) NOT NULL,
[dataflow_id] [varchar](100) NOT NULL,
[workflow_id] [varchar](100) NOT NULL,
[output_id] [varchar](100) NOT NULL
) ON [PRIMARY]
GO
Field reference
Field name | Description |
---|---|
| This is an identity column that will be unique for all records in this table. |
| This is the name of the promotion that the codes are associated to. This will be used to define a view to support using as the source of the metadata values. |
| This is the coupon code itself. |
| Indicates if it is a Group or Individual coupon code. |
| This is the RPI Resolution Key field name for the audience being used with the coupon assignment process. |
| This is a placeholder for an integer type resolution key. It can be left with this default name or be renamed to match the name of the resolution key that will be loaded to the field. |
| Additional integer-based resolution key field if coupons need to be assigned using more than one resolution. |
| Additional integer-based resolution key field if coupons need to be assigned using more than one resolution. |
| This is a placeholder for a string type resolution key. It can be left with this default name or be renamed to match the name of the resolution key that will be loaded to the field. |
| Additional string-based resolution key field if coupons need to be assigned using more than one resolution. |
| Additional string-based resolution key field if coupons need to be assigned using more than one resolution. |
| Dataflow ID that is in the temp table when the coupon project is executed. |
| Dataflow ID that is in the temp table when the coupon project is executed. |
| Dataflow ID that is in the temp table when the coupon project is executed. |
Promotion code view
The promotion code view is used to support dynamically updating the list of promotion codes to be selected in RPI when setting the metadata. As new promotion codes are loaded, they will be available for selection in RPI.
Replace <RPI Primary Database Name>
with the name of the primary RPI Database.
USE <RPI Primary Database Name>;
GO
SET ANSI_NULLS ON;
GO
SET QUOTED_IDENTIFIER ON;
GO
CREATE VIEW [dbo].[RPI_UNIQUE_COUPON_PROMOTION_CODE] AS
SELECT DISTINCT Promotion_Code
FROM <RPI Primary Database Name>.[dbo].[RPI_COUPON_POOL];
GO
Appendix B: Stored procedures used by the coupon assignment project
sp_RedPoint_ForceWaitDM
This Stored procedure forces the RPDM job to pause for a duration of time so that you can attach to the project when debugging or modifying the RPDM project and want to watch it execute.
CREATE PROCEDURE [dbo].[sp_RedPoint_ForceWaitDM]
@outvar int OUTPUT,
@retryDelay char(8) = '00:00:00'
AS
BEGIN
DECLARE @ReturnInfo varchar(255);
-- Validate the @retryDelay parameter
IF ISDATE('2000-01-01 ' + @retryDelay + '.000') = 0
BEGIN
SELECT @ReturnInfo = 'Invalid time ' + @retryDelay + ', hh:mm:ss, submitted.';
PRINT @ReturnInfo;
RETURN(1);
END
-- Delay execution for the specified time
WAITFOR DELAY @retryDelay;
-- Set output variable
SELECT @outvar = 1;
END
sp_RPI_CouponProject_Lock
This stored procedure is used to see if it can lock the project for execution. If it fails to get a lock, it will delay and retry a configurable number of times before failing the interaction. If the project is not being run at the time this procedure is executed or after a retry, it will lock the project by updating the coupon_project_runstatus
table with a status of running
. At that point, the coupon project will run and assign the appropriate coupons.
CREATE PROCEDURE [dbo].[sp_RPI_CouponProject_Lock]
@result int OUTPUT,
@numRetries int,
@retryDelay char(8) = '00:00:00'
AS
BEGIN
DECLARE @ReturnInfo varchar(255);
-- Validate the @retryDelay parameter
IF ISDATE('2000-01-01 ' + @retryDelay + '.000') = 0
BEGIN
SELECT @ReturnInfo = 'Invalid time ' + @retryDelay + ', hh:mm:ss, submitted.';
PRINT @ReturnInfo;
RETURN(1);
END
SET NOCOUNT ON;
DECLARE @lockCheck int = 0;
-- Check for idle status with lock_id = 1
SELECT @lockCheck = COUNT(*)
FROM coupon_project_runstatus WITH (TABLOCKX)
WHERE lock_id = 1 AND STATUS = 'idle';
-- If lock is available, update the status to 'running'
IF @lockCheck = 1
BEGIN
UPDATE coupon_project_runstatus
SET STATUS = 'running'
WHERE lock_id = 1;
SET @result = @lockCheck;
END
ELSE
BEGIN
DECLARE @retry int = 1;
-- Retry mechanism if lock is not available
WHILE @retry <= @numRetries
BEGIN
-- Wait for the specified delay
WAITFOR DELAY @retryDelay;
-- Increment retry counter
SET @retry = @retry + 1;
-- Check lock status again
SELECT @lockCheck = COUNT(*)
FROM coupon_project_runstatus WITH (TABLOCKX)
WHERE lock_id = 1 AND STATUS = 'idle';
-- If lock becomes available, update status and exit loop
IF @lockCheck = 1
BEGIN
UPDATE coupon_project_runstatus
SET STATUS = 'running'
WHERE lock_id = 1;
SET @result = @lockCheck;
BREAK;
END
END
END
-- Set the final result
SET @result = @lockCheck;
END;
GO
coupon_project_runstatus
This is the table that is used to support the locking of the project to prevent concurrent execution.
CREATE TABLE [dbo].[coupon_project_runstatus] (
[lock_id] [int] NOT NULL,
NOT NULL,
CONSTRAINT [PK_coupon_project_runstatus] PRIMARY KEY CLUSTERED
(
[lock_id] ASC
)
WITH (
PAD_INDEX = OFF,
STATISTICS_NORECOMPUTE = OFF,
IGNORE_DUP_KEY = OFF,
ALLOW_ROW_LOCKS = ON,
ALLOW_PAGE_LOCKS = ON
) ON [PRIMARY]
) ON [PRIMARY];
GO
-- Insert an initial record into the table
INSERT INTO [dbo].[coupon_project_runstatus] ([lock_id], [status])
VALUES (1, 'idle');
GO
Appendix C: RPI to RPDM integration configuration
This section provides some details on integrating RPI with RPDM.
There is more extensive documentation on setting up and using Data Process Projects that provides additional details on configuration and the additional ways that Data Process Projects can be used.
Here are some notes on integrating RPI with RPDM. This integration is required for several RPDM-dependent features in RPI such as:
Data Projects (Data Intake): supports importing flat files into RPI warehouse
Data Process Projects: supports running Data Management projects during the execution of an interaction workflow and/or interaction audience to augment RPI capabilities (e.g., couponing)
Channel States Import: leverages RPDM to facilitate import of channel execution fulfillment states results, most used in environments where high volume of results are generated
Calling RPDM from RPI requires RPDM Operational API (OAPI). The OAPI Download Center can be found here (direct links to the software download and the installation guide).
To configure RPI to run RPDM jobs using the DM Operational API web service configure the following settings in RPI:
DataManagementcredentials
: set the credentials for connecting to RPDMDataManagementExecutionServer
: leave this value blankChange
DataManagementIntegrationType
from0
to1
Configure parameter
DataManagementOAPIWebServiceAddress
to point to the base URL for the OAPI web serviceDataManagementServerName
: set to the RPDM site server hostnameDataManagementServerName
: set to the site server port (normally40420
for RPDM v9)
Example configuration:
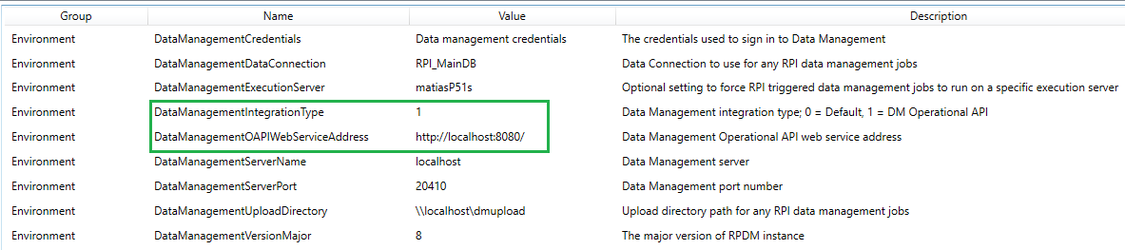
Appendix D: Create the view for coupon resend
This is the statement to create the view for the coupon resend:
/******
Object: View [dbo].[RPI_COUPON_ASSIGNMENT_RESEND]
Notes: By having an inner join to Offer History this will
ensure that any Coupon assignments that have been
Rolled back will not be eligible for resend
******/
SET ANSI_NULLS ON;
GO
SET QUOTED_IDENTIFIER ON;
GO
CREATE VIEW [dbo].[RPI_COUPON_ASSIGNMENT_RESEND] AS
SELECT
CA.[coupon_assignment_id],
CA.[promotion_code],
CA.[coupon_code],
CA.[coupon_type],
CA.[RPIResolutionKey],
CA.[resolution_key_int01],
CA.[resolution_key_int02],
CA.[resolution_key_int03],
CA.[resolution_key_str01],
CA.[resolution_key_str02],
CA.[resolution_key_str03],
CA.[insert_ts],
CA.[dataflow_id],
CA.[workflow_id],
CA.[output_id],
P.[FirstName],
P.[LastName],
P.[PID],
P.[EmailAddress],
OH.OfferActivityName
FROM
[RPIQASampleTenant].[dbo].[RPI_COUPON_ASSIGNMENT] CA
INNER JOIN
Person P ON P.PID = CA.[resolution_key_int01]
INNER JOIN
[dbo].[OfferHistory] OH ON CA.[resolution_key_int01] = OH.[PID]
AND CA.[dataflow_id] = OH.[DataflowID]
AND CA.[workflow_id] = OH.[WorkflowID];
GO
Appendix E: RPDM error
Data process project errors occur. This error is an indication that something is misconfigured in the RPDM project. In this case, the ProspectiveBuyerKey
was spelled incorrectly in the RPDM job, so it was not able to select the field from the Dataflow table, and this is the error that it threw.
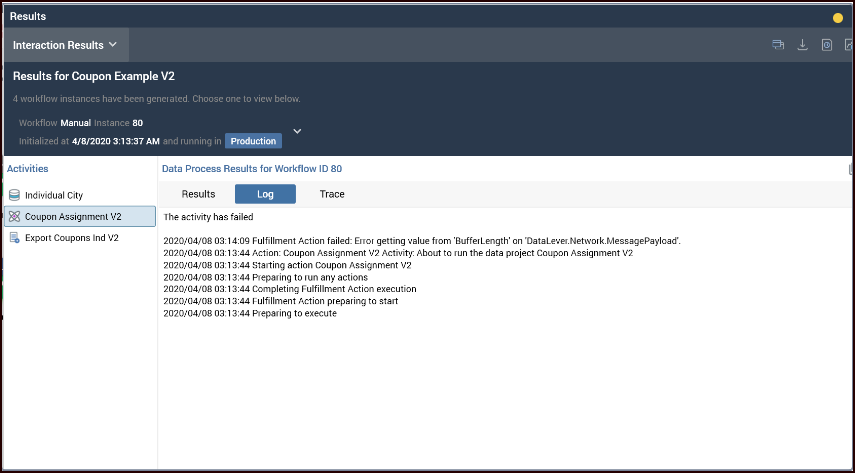
Appendix F: RPDM repositories for supporting coupons
In this appendix, the various RPDM repositories for supporting coupons will be saved.